Rich Internet Applications (RIA) are created by manipulating the Document Object Model (DOM) elements in various ways. Before working on DOM elements we need to have an efficient and easy way to identify and select the elements required. The JQuery library provides different options to identify an element. The power of JQuery is that it allows us, users of the library, to write our own selectors.
All selectors will start with a $ symbol and the selector string is placed within (“). Note that $ is just a short form of JQuery. All selectors will return an array of elements that satisfy the condition. An element in the array can be indexed as a JavaScript array or by using “get”, the JQuery utility method. For example, to get the first DOM element in the page, we can use both $('*')[0]
and $('*').get(0)
.
Basic selectors
The most efficient way of selecting an element is by using the ID of that element. JQuery, in turn, uses the native method, getElementById()
. For example, to get an element with the ID msg, the selector should be $('#msg')
.
An element can be selected using its type. To select the entire span element in a page, we can use $('span')
. For an appealing look and feel for an element, it should be associated with a class. JQuery provides ways to select elements based on this class. For example, when we need to associate a click operation with all the elements that have a class .button
, then…
$('.button').click(function() { console.log("Element with class button is clicked"); });
$('*')
is used to select all the DOM elements in a page.
We can combine different selectors together using the comma. The resulting array will contain all the elements that satisfy any of the condition(s) passed. For the following selector query:
$('#msgdiv , .msgdiv')
< …the resulting array will contain elements that have their class set to msgdiv or an element that has an ID equal to msgdiv. The same set of elements can also be matched using the JQuery function add().
$('#msgdiv').add('.msgdiv')
Form selectors
Form selectors help us to select an element based on its type. Though the same result could be achieved using an attribute selector (as we will see), the form selector provides a shorter way. As an example, :button
will select all the <input type="button">
elements. The following table lists all form selectors.
Selector | Function | Examples |
:hidden, :text, :password, :checkbox, :image, :file, :submit, :reset, :radio | To select all the corresponding <type> elements, <input type=<type>> | $(‘:hidden’) will select all the hidden elements |
:input | To select all the input elements like text area | $(‘:input’) |
:button | Will select all the button elements that include <input type=”button”>, <input type=”submit”> and <input type=”reset”> |
Form filters also help us in making a selection based on the state of a particular element, like enabled
, selected
, etc, as shown in the following table.
Filter | Function | Examples |
:enabled | All elements in an enabled state | $(‘:enabled’) |
:disabled | All elements that are in a disabled state | $(‘:disabled’) |
:checked | Radio buttons and check boxes that are checked | $(‘:checked’) to select all the radio buttons/check boxes that are selected by the user |
:selected | Option elements that are selected | $(“select option:selected”) will find the currently selected option |
The power of form selectors can be understood when two or more of them are combined. For example, $(':radio:checked')
will select the radio buttons that are selected by the user.
Hierarchy selectors
These selectors are used to select elements based on their positions with respect to other elements. There are four ways, using which we will be able to select an element based on its hierarchy in the DOM. Let’s look at the HTML snippet example shown in Figure 1 to explain hierarchy selectors. The following table lists hierarchy selectors.
Selector | Function | Examples (as per the HTML snippet in Figure 1) |
$(parent child) | To select all the child elements that are under the specified parent. The child can be directly under the parent or can also be the grand child of the parent | $(‘#upperul li’) will select all the four li(s). Two directly under UL and two under innerul. |
$(parent > child) | To select all the child elements that are ‘directly’ under the specified parent | $(‘#upperul > li’) will select only the first two li elements. Since the next two li elements are not directly under #upperul |
$(previous ~ next) | To select all the next elements (only siblings) that are after the previous element | $(‘:text ~ :button’) will select both the buttons |
$(previous + next) | To select all the next (only siblings) elements that are placed ‘immediately’ after the previous element | $(‘:text + :button’) will select only the first button element |
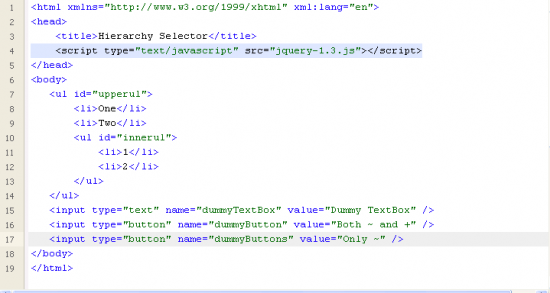
Filters
Filters are used to select a subset of elements from a set of elements. These filters are extremely useful when combined with the selectors that we have seen previously.
Basic filters
These filters are useful when we need to select an element based on its position in the returned array. We have already seen some ways to do this—for example get(n) and [n] will give the nth element from the returned set. But get(n) or [n] can’t be used in the chaining of functions. Filters (that we are going to discuss) will return a JQuery object that can be used in chaining, like $(':button:first').addClass("chained")
.
In our zebra table example, seen in the last article [published in the May 2009 issue of LFY] we have given different colours to every other row in a table. The same can be achieved using the odd or even selector. tr:odd
will select every other odd element on a page, whereas tr:even will select every other even element on a page. In our zebra table example, we have used "$(".lfy tr:even").addClass("alt");"
to select every even row under the table that has class lfy
and added a class alt
to them. The following table summarises the list of basic filters and their functions with an example.
Filter | Function | Examples |
:first | To select the first element on the matched set | $(‘:button:first’) will return the first button element in the page |
:last | To select the last element on the matched set | $(‘:button:first’) will return the last button element in the page |
:even | To select the even elements on the matched set | $(‘#timetable tr:even’) select all the even rows under table with id timetable |
:odd | To select the odd elements on the matched set | $(‘#timetable tr:odd’) select all the odd rows under table with id timetable |
:lt(n) | To select the elements that are before the nth position, excluding n | $(‘*:lt(2)’) will return the first 2 elements |
:gt(n) | To select the elements that are after the nth position excluding n | $(‘*:gt(5)’) will return all the elements except the first 5 |
:eq(n) | To select the element in the given nth position | $(‘:eq(5)’) will return the 5 elements |
Visibility filters
These filters are used to select elements based on their visibility in the page. An element can be in a visible or hidden state. For example, to select all the visible elements in a page, we can use $(':visible')
. $(':hidden')
will select all the hidden elements in a page. If we analyse the previous selector syntaxes, we would know that, by default, all filters will take $('*')
as a matched set when none is explicitly mentioned. The following table lists the visibility filters and their functions.
Filter | Function | Examples |
:visible | All elements that are not selected as part of the :hidden filter | $(‘:visible’) to select all the elements that are visible |
:hidden | All elements that are hidden either by the type or through CSS | $(‘:hidden’) to select all the elements that are hidden |
Child filters
These filters are used to select an element based on its position with respect to its containing element, known as the parent. When we need to select the first rows from all the available tables in a page, we can use $('tr:first-child')
. To make this selector work on a particular element (table), we need to prepend the selector with that element. For example, $('#tableid tr:first-child')
will select the first row from the table with ID tableid
. As we saw in basic filters, here too we have the nth child (number/odd/even). For example, $('#tableid td:nth-child(odd)')
will select all the odd columns from the page.
We can use the :only-child
selector to select the element that is the ONLY child for its parent.
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en"> <div></div> <div> <div><span>MySpan1</span><button>MyButton1</button></div> <div><span>MySpan2</span></div> </div> </html>
In the above HTML snippet, $('span:only-child')
will select the span with value MySpan2
. The following table gives the complete list of child selectors with their usage patterns.
Filter | Function | Examples |
parent>child | All children of the parent | $(‘table>tr’), matches all the table rows |
:first-child | To select any element that is the first child for its parent | $(‘#tableid tr:first-child’), matches the first row from the table with ID tableid |
:last-child | To select all the elements that are the last children for its parents | $(‘#tableid tr:last-child’), matches all that’s in the last row from the table with ID tableid |
:nth-child(n/odd/even) | To select a particular row using its position within the parent. Can be group-based and be odd or even | $(‘table td:odd’) to select all the odd columns from all the available tables |
Attribute filters
Attribute filters are used to select elements based on the attribute values. For example, the text box <input type="text" name="dummyTextBox" value="Dummy TextBox" />
can be selected in the following way:
Filter | Function | Examples |
= | Equal to | $(‘:input[name=dummyTextBox]’), matches the input element that has the name equal to dummyTextBox |
!= | Not equal to | $(‘:input[name!=someothername]’), matches all the input elements that don’t have name attributes or name attributes of value other than someothername |
*= | Contains | $(‘:input[name*=dummyTextBox]’), matches all the elements that contain dummyTextBox in the value of a name attribute |
^= | Starts with | $(‘:input[name^=dummyTextBox]’), matches all elements whose name attribute value starts with dummyTextBox |
$= | Ends with | $(‘:input[name$=dummyTextBox]’), matches all elements whose name attribute value ends with dummyTextBox |
Content filters
Content filters are used to select elements based on what the element contains. For example, :contains(text)
helps us to select elements that contain the given text. To explain ‘content filter’, let’s take the HTML snippet shown in Figure 2.
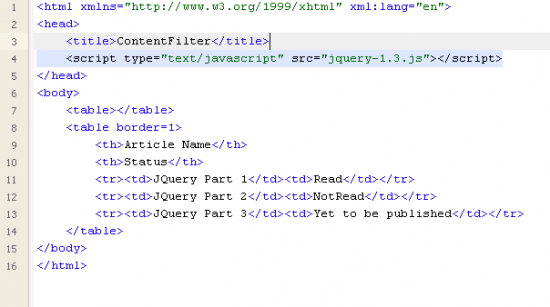
The following table lists the various content filters and their functions.
Filter | Function | Examples |
:contains (text) | To select all the elements that have the text specified. Case sensitive searches will be made | $(‘td:contains(Read)’), will select the second column from the first two rows. $(‘td:contains(read)’) won’t select any element |
:has(p) | To select all the elements that satisfy the selector criteria passed | $(‘table:has(td)’), this will select only the second table |
:empty | To select all the elements that have no children | $(‘table:empty’), will select the first table. It’s not that this also includes text node; it means $(‘td:empty’) will select all columns that don’t have any value |
:parent | To select all elements that have child nodes | $(‘table:parent’), will select the second table |
Note that if content filters are made to filter on * then they will select the complete DOM, even if only one DOM element satisfies. For example, $('table:has(td)').size()
will be 1, but $(':has(td)')
will be of size 7 (in the above fragment) since it selects all the above tr
(s), the body and HTML too.
Not selectors
We have looked at a good number of selectors till now. In certain scenarios, when we need a function that will negate the available functionality, the :not
selector comes to the rescue. As we know, we can use $('checkbox:checked')
to get all the check boxes that are selected. But how about getting the check boxes that are not selected by the user? It’s simple! We need to use :not
in front as $('checkbox:not('checked'))
.
Selector commands
There are a set of JQuery commands/functions that can be used to select an element. We know that every JQuery selector will return an array of elements. To get a particular element out of the array, we can use get(number)
. So $(':button').get(0)
is equivalent to $(':button:first')
. The inverse of get is index, which is used to find the position of an element in an array of elements. Considering a page that has only one submit, element $('*').index($(':submit')[0])
will return the position of the submit button in the complete DOM. When we need to select all input elements that are either of type radio or check box then we can use any one of the following: $(':radio,:checkbox')
or $(':radio').add(':checkbox')
. Following which, the add(JQuery expression)
will add the result to the wrapped set.
When we need to remove the element that is added as part of the previous command from the wrapped set, then we can use end()
. For example:
$(':image').add(':text').addClass('inputandtextclass').end().add('imageclass')
In the above case, the inputandtextclass class is applied to both image and text elements, whereas imageclass class will be applied to the image elements only.
We use find(expression)
to select elements that match the given criteria and also the descendants of the already selected element set. We use filter(expression)
to select elements that match the given criteria from the given set.
<div> <button value="Check">Check</button> </div>
In the above HTML snippet, to select the button using:
- find, use
$('div').find(':button')
. This will search for buttons under div element. - filter, use
$('div :button').filter(':button')
. This will search for the button element in the first wrapped set, which has both div and button.
Although we have equivalent selectors $('div button')
and $(':button',$('div'))
, respectively, find and filter will be more useful in chaining.
JQuery selectors and filters have made the selection of a DOM element easier. It is always better to use the ID selector since JQuery uses the browser native method getElementByID()
. So in any case, the ID selector is much faster than other selectors. Moreover, it’s always better to cache the result of selectors. The cached results can be used (if the DOM element is not updated dynamically) for future purposes, thereby making the response faster. To practice the above concepts, click codylindley.com/jqueryselectors.
aaaaaaaaaaaaaaaaaa aaaaaaaaaaa aaaaaaa