To get started, you need to install the framework (I assume you already have a working Web and database server set up). Download the zip file from here; extract it, and move the folder to your Web server’s DocumentRoot (usually /var/www/
).
While moving it, I renamed the extracted codeigniter
folder to ci2
to save on typing. Now, in a browser, visit http://localhost/ci2/
. If you see something like what’s shown in Figure 1, CodeIgniter has been successfully installed.

The page has a user guide link for help, if you need it. CodeIgniter also has a very huge and supportive online forum community.
MVC
CodeIgniter follows the MVC (Model-View-Controller) software architecture for development. View and Controller are necessary, while Model is optional. MVC’s greatest strength lies in the fact that it divides the application. If you need to change database queries, you don’t touch other files, and vice-versa.
Listed below are the files that MVC is made up of:
Model: All database-related work is done through the model file.
View: The frontend view (here, HTML) design is contained in the view file.
Controller: The Controller, the base of MVC, controls everything inside the application. You can use Views and Models only through a Controller; without a Controller file, your CodeIgniter app will not run.
The application
subdirectory is your main directory, so let that be your main focus.
Configuration
To avoid runtime errors, you need to do some CodeIgniter configuration changes in three files — autoload.php
, config.php
and database.php
in the config
subdirectory.
As per the lines of code shown below, locate and make the changes in the corresponding file, adjusting for your local setup.
database.php
(database-related configuration):$db['default']['hostname'] = 'localhost'; # Server hostname. $db['default']['username'] = 'databaseusername'; #Your DB account username. $db['default']['password'] = 'databasepassword''; #Database password. $db['default']['database'] = 'databasename'; #Database name. $db['default']['dbdriver'] = 'mysql'; #Database driver
autoload.php
: This file automatically loads parameters at program start, saving you from having to constantly import these.$autoload['libraries'] = array('database'); #auto-load database library; others are session, email etc. $autoload['helper'] = array('url','form'); #helper API (see below).
Helpers are impressive APIs that speed up your work. There are many, like
file
,form
, etc. We will talk about them later. If you want to learn more about helpers, refer to the user guide.config.php
(a general configuration file):$config['base_url'] = 'http://localhost/ci2/'; #Default CodeIgniter URL $config['index_page'] = 'index.php'; #Default index page.
Let’s roll
So, if you are all set, let’s start with the traditional “Hello World”. Let’s create the main, required Controller file as hello.php
, in the application/controllers subdirectory, with the following code:
// hello.php <?php class Hello extends CI_Controller { #1 function index() { #2 echo "Hello World"; } } ?>
Let’s understand this code. The class name should have an initial uppercase letter (e.g., Hello as above) and ideally, should match the PHP file name. You must extend the CI_Controller
class to associate your file to the URI (Uniform Resource Identifiers).
The function index()
, containing your simple code, is the default function that is executed. Now visit http://localhost/ci2/index.php/hello
in your browser, to see “Hello World” echoed.
abc()
, the URL would be http://localhost/ci2/index.php/hello/abc
.Database test
Let’s write a demo program to test database connectivity, in which you can create a table, insert records, fetch the records and display them in an HTML table. Let’s use all three files, Model, View and Controller here; and create them with the exact paths given for each.
Here is the model file — /application/models/dbtest.php
:
<?php class DbTest extends CI_Model { function createTable() { $query="create table lfy(article varchar(50), author varchar(50))"; $this->db->query($query); } function insert() { $insert=array('article'=>'CodeIgniter Part 1', 'author'=>'Ankur Aggarwal'); $this->db->insert('lfy',$insert); } function showAll() { $fetch=$this->db->get('lfy'); $fetch=$fetch->result_array(); return $fetch; } } ?>
Let’s understand the code. Our DbTest
class extends the CI_Model
class; this is needed to use certain APIs. The createTable()
function creates the lfy
table with varchar
columns article
and author
. Use the query method of the db instance member to run the SQL query. The insert()
function creates an associative array whose key is the column name, and value is the data we want to insert.
The db->insert
method takes the table name and the data array as arguments. The db->get call is equivalent to a SELECT * FROM
SQL query. You can also pass an ORDER BY
value like ASC
as the second (optional) argument. Now fetch results into an array and return it.
Here is the Controller file — /application/controllers/dbtestcontroller.php
:
<?php class DbTestController extends CI_Controller { function index() { $this->load->model('dbtest'); #1 $this->dbtest->createTable(); #2 $this->dbtest->insert(); $data['records']=$this->dbtest->showAll(); #3 $this->load->view('dbtestview',$data); #4 } } ?>
To use the Model and View in the Controller, you must load them, giving the file base name as the argument. Load the dbtest
model, call its createTable
and insert()
functions, then assign the showAll()
returned array to a records
attribute of the $data
variable, which you pass into the (loaded) view file. The records
attribute will be accessible as a $records
variable in the view file.
Here is the View file — /application/views/dbtestviews.php
:
<html> <head> <title>Db Test </title> <meta charset='utf-8'> </head> <body> <pre> <?php echo "<table border=5><tr><td>Article</td><td>Author</td></tr>"; foreach($records as $row) { echo "<td>".$row['article']."</td><td>".$row['author']."</td></tr>"; } echo "</table>"; ?> </pre> </body> </html>
The above code is quite simple. After creating all three files in the browser, visit http://localhost/ci2/index.php/dbtestcontroller
. You should see something like what’s shown in Figure 2.
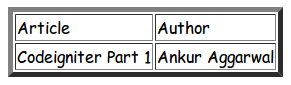
There are a lot of APIs in CodeIgniter that will speed up your development. Read up on it! I hope you will enjoy working with it.
In the next part of this series, we will explore “form” helpers, form validations, database checks and a little about tables. Feedback, suggestions, queries are always welcome!
I tried it but could not run even the simple hello.php file..:(
it shows the following error:
Not Found
The requested URL /ci2/index.php/hello/ was not found on this server.
Here is the Controller file — /application/controllers/dbtestcontroller.php:
1234567891011<?phpclass DbTestController extends CI_Controller { function index() { $this->load->model(‘dbtest’); #1 $this->dbtest->createTable(); #2 $this->dbtest->insert(); $data[‘records’]=$this->dbtest->showAll(); #3 $this->load->view(‘dbtestview’,$data); #4 } }?>
Thr’s a slight mistake of spelling where it does not connect to “dbtestview” –> Add “s”=====> dbtestviews
It works out super !
Thanks Ankur :)
[…] 8. Let’s Play With CodeIgniter Part 1 : Teaches you the basics of the PHP based framework ‘CodeIgniter’ . This frameworks is really useful and changes the way I used to code in PHP. It follows the MVC approach. It was published in the Oct Edition. Click To Read […]
good work buddy…
got the concept about MVC now…
I want perform with CodeIgniter because it is fast, efficient, lightweight and more able and also it has an excellent performance as well as presentation.