Deep learning has impressive data learning and prediction capabilities, making it very useful for a wide range of industries. TensorFlow is a popular open source library for deep learning applications because it is versatile, scalable, and can integrate with other tools. This article demonstrates how to implement a convolutional neural network (CNN) model with TensorFlow.
When it comes to developing and training machine learning models, TensorFlow is an extremely useful and versatile deep learning library. Its popularity and large community also make it a good choice for those looking to learn and apply deep learning in their work. Figure 1 shows several reasons why TensorFlow is a popular choice for deep learning frameworks.
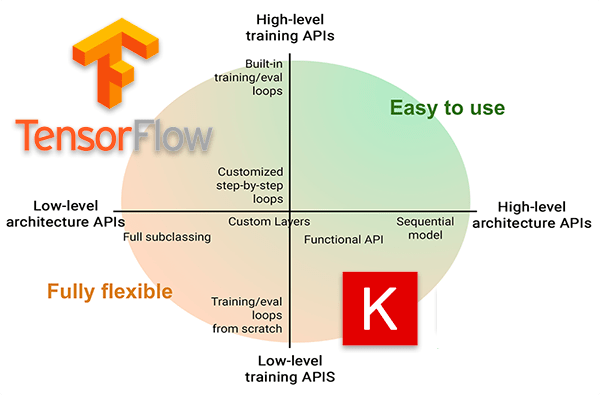
Comparison of TensorFlow, PyTorch, and Keras
Three widely used deep learning frameworks are Keras, TensorFlow, and PyTorch. Keras’ application programming interface (API) is a set of high-level building blocks that is intuitive, flexible, and easy to use. TensorFlow provides a low-level interface to create a neural network and high-level API (such as Keras) for easy and efficient model building. PyTorch’s dynamic computational network enables more flexible and efficient model construction than TensorFlow.
Table 1 presents a comparison of these three deep learning frameworks. Overall, each framework has its own set of strengths and drawbacks, and their selection is determined by the use case.
Key programming elements in TensorFlow
Tensors, variables, and placeholders are the key programming elements in TensorFlow, making it a powerful platform.
Tensors: Tensors are TensorFlow’s fundamental data structure. They are multidimensional arrays, similar to NumPy arrays, but with additional features such as support for GPU acceleration and automatic differentiation. In the TensorFlow library, a tensor can be created using the following command:
import tensorflow as tf # Creating a tensor a = tf.constant([5, 7]) print (a)
Variables: Parameters of a model, such as a neural network’s weights and biases, are stored and updated in variables. They are created using the tf.Variable() function, which takes an initial value as its argument. The following command can be used to create variables in TensorFlow:
import tensorflow as tf # Creating a variable b = tf.Variable(23) print (b)
Placeholders: When training or inferencing a TensorFlow model, placeholders are frequently used to inject input data into the model. You can use the tf.placeholder() function to create a placeholder in TensorFlow. This function takes two arguments: the data type of the tensor that will be fed into the placeholder and the shape of the tensor (which can be optional). Here is an example of creating a placeholder for a 2D tensor of floats:
import tensorflow as tf # create a placeholder for a 2D tensor of floats x = tf.placeholder(tf.float32, shape=(None, 2))
Criteria | TensorFlow | PyTorch | Keras |
Developed by | Meta (Facebook) | ||
Written in | C++, Python, CUDA | Lua | Python |
Compatibility | Large data set | Large data set | Small data set |
Debugging capability | Difficult | Good | Not required |
Choice of use | Scalability and performance | Flexibility and dynamic nature | Ease of use and simplicity |
Popular industry use cases | Image classification, language translation, speech recognition, etc |
Computer vision, NLP, recommendation system, etc | Speech recognition, healthcare, sentiment analysis, text classification |
Implementing a CNN deep learning model using TensorFlow
A CNN deep neural network is frequently utilised for image categorisation, object detection, and other computer vision applications. Figure 2 shows the Python code example executed on Google Collab notebook for implementing a CNN deep learning model using TensorFlow.
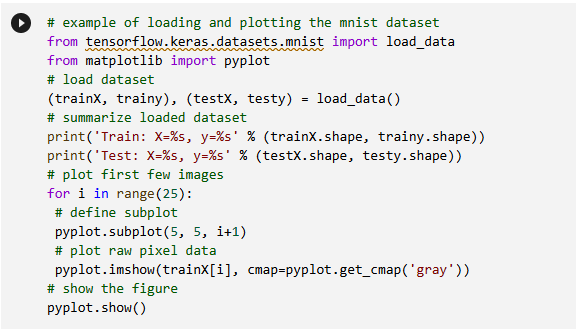
We have trained the CNN model using the MNIST data set in this example. In the machine learning community, the MNIST data set is frequently used as a benchmark for image classification tasks, notably for assessing the performance of deep learning models like CNNs. It is a popular data set due to its simplicity and accessibility, and has been used for various applications including handwriting recognition, digit classification, and optical character recognition.
The output of our implemented CNN deep learning model using the MNIST data set is shown in Figure 3. The data set consists of 70,000 images, each of which is a grayscale image of a handwritten digit from 0 to 9. The images are 28×28 pixels and centred within a 32×32-pixel image.
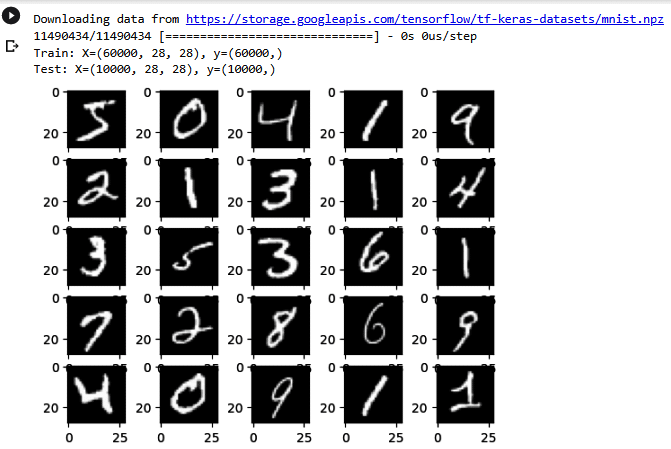
TensorFlow is a Google open source software library. It was designed for tasks requiring complex numerical computations, and is popular because it supports Python and C++ API. It has faster compile times and supports CPUs as well as GPU distributed processing. Overall, TensorFlow’s flexibility, scalability, and facilitation of neural network architectures make it well-suited for various industry applications.