Since we’re discussing sockets in network programming, newbies should first understand the OSI model layers, and the protocols used in these layers. Every layer in the model is responsible for doing some particular work, to make data transfer possible on the network. Each layer abstracts the lower layers’ work to the layer above it. I recommend that if you don’t know the ISO OSI (Open Systems Interconnection) Reference Model, you should read up on it. A good starting point is Wikipedia.
Here, we focus on the session layer (which establishes and maintains sessions) and the transport layer, which provides end-to-end reliable and unreliable delivery. Some protocols are TCP (for reliable connections), UDP (non-reliable connections) and SCTP (an advanced protocol with multiple-connection capability). Please also look at this and this for information on TCP/IP.
Transmission Control Protocol (TCP)
TCP is a connection-oriented protocol that provides a reliable, full-duplex byte stream to users. Here, we will directly communicate with the transport layer, using TCP from the application layer, where users can communicate with the program.
Here are some important features of TCP. It is a reliable protocol (the opposite of connection-less UDP, which we will look at in later articles). After transmitting a packet, it awaits an acknowledgement; if one is not returned, it retransmits the packet a number of times (depending upon the implementation). If the data cannot be transmitted, it notifies the user and closes the connection.
TCP decides how long to wait for an acknowledgement by the estimating RTT (Round Trip Time) between server and client. It also associates sequence numbers with segments, so that segments arriving out of order can be reordered at the receiver. Duplicate segments (retransmitted due to delays) can thus also be ignored. TCP provides flow control; the receiver can tell the sender how many bytes it is willing to receive, so that a slow receiver is not flooded.
TCP connections are full-duplex — the application can both send and receive data simultaneously.
Simple servers
Now let’s create a simple server to understand Internet sockets (server.c
). Initially, our code will be for IPv4, but in later articles, we’ll look at IPv6, and then move to protocol-independent code.
#include <stdio.h> #include <unistd.h> #include <sys/types.h> #include <sys/socket.h> #include <netinet/in.h> int main() { int sfd, cfd; int ch='k'; struct sockaddr_in saddr, caddr; sfd= socket(AF_INET, SOCK_STREAM, 0); saddr.sin_family=AF_INET; /* Set Address Family to Internet */ saddr.sin_addr.s_addr=htonl(INADDR_ANY); /* Any Internet address */ saddr.sin_port=htons(29008); /* Set server port to 29008 */ /* select any arbitrary Port >1024 */ bind(sfd, (struct sockaddr *)&saddr, sizeof(saddr)); listen(sfd, 1); while(1) { printf("Server waitingn"); cfd=accept(sfd, (struct sockaddr *)NULL, NULL); if(read(cfd, &ch, 1)<0) perror("read"); ch++; if(write(cfd, &ch, 1)<0) perror("write"); close(cfd); } }
About the code
The first thing to look at is struct sockaddr_in
. This structure is used to hold an Internet (IP) address in the sin_addr
member, which is of the type struct in_addr
and holds a 32-bit unsigned integer. The port number is held in the sin_port
member, an unsigned 16-bit integer (so port numbers must be less than 65536).
Next, let’s look at a call to socket()
(the includes
required are sys/types.h
and sys/socket.h
):
int socket(int domain, int type, int protocol);
A successful call to socket()
returns a descriptor that will be used for end-point communication. The first argument, domain
, specifies a communication domain — the protocol family to be used for communication. According to sys/sockets.h
, these are:
Name | Purpose |
AF_UNIX, AF_LOCAL | Local communication |
AF_INET | IPv4 Internet protocols |
AF_INET6 | IPv6 Internet protocols |
AF_IPX | IPX — Novell protocols |
AF_NETLINK | Kernel user interface device |
AF_X25 | ITU-T X.25 / ISO-8208 protocol |
AF_AX25 | Amateur radio AX.25 protocol |
AF_ATMPVC | Access to raw ATM PVCs |
AF_APPLETALK | AppleTalk |
AF_PACKET | Low-level packet interface |
AF stands for Address Family. We’re using AF_INET
here — Internet IPv4. The next argument, type
, specifies the type of communication, from these choices:
SOCK_STREAM | Sequenced, reliable, two-way, connection-based byte stream (TCP,SCTP, etc) |
SOCK_DGRAM | Datagrams — connectionless, unreliable (UDP) |
SOCK_SEQPACKET | Sequenced, reliable, two-way, connection-based data transmission path for datagrams of fixed maximum length (SCTP) |
SOCK_RAW | Raw network protocol access (Transport layer protocols not required) |
The protocol
argument specifies a protocol to be used with the socket. Normally, only a single protocol exists to support a particular socket type within a given family (as specified within parentheses above). In such cases, this argument is 0.
Next, let us set the address in sin_addr
, as explained above. With the call to socket()
, a socket is created, but no address is assigned to it. So we have the bind()
function:
int bind(int sockfd, const struct sockaddr *addr, socklen_t addrlen);
This is used to bind the socket descriptor sockfd
with the address addr
; and addrlen
is its length. This is called assigning a name to the socket. Next up is listen()
:
int listen(int sockfd, int backlog);
The listen()
call marks the socket referred to by sockfd
as a passive socket — one that will be used to accept incoming connections. The socket type should be SOCK_STREAM
or SOCK_SEQPACKET
, i.e., a reliable connection. The backlog
argument defines the maximum length of the queue of pending connections for sockfd
. If the queue grows above this, connections will be refused by clients.
Next, let’s enter an infinite loop to keep on serving the client requests. Here we have to create another socket descriptor for the client, by calling accept
. Now, whatever is written to this descriptor is sent to the client, and whatever is read from this descriptor is the data the client sent to the server:
int accept(int sockfd, struct sockaddr *addr, socklen_t *addrlen);
The accept()
system call is used with socket types SOCK_STREAM
and SOCK_SEQPACKET
. It extracts the first connection request on the queue of pending connections for the listening socket sockfd
, creates a new connected socket, and returns a new descriptor referring to that socket — in our program, cfd
.
The newly created socket is not in the listening state. The original socket sockfd
is unaffected by this call. The addr
argument contains the address of the remote machine to which we connect; since we don’t know the client’s address in advance, it’s NULL here. The addrlen
argument is the length of addr
— so, again, this is NULL.
Then, let us read a character (sent by the client to the server) from the descriptor with read()
, increment it, and write to the descriptor using write()
, to send the character to the client. Then close the descriptor by calling close()
.
The error handling I chose is based on the knowledge that these functions return a negative number on failure; I use perror()
to display an error number message.
The client
And now the client (client.c
). This client sends a character to the server running on port 29008 (or any arbitrary port):
#include <stdio.h> #include <unistd.h> #include <sys/types.h> #include <sys/socket.h> #include <netinet/in.h> int main(int argc, char* argv[]) { int cfd; struct sockaddr_in addr; char ch='r'; cfd=socket(AF_INET, SOCK_STREAM, 0); addr.sin_family=AF_INET; addr.sin_addr.s_addr=inet_addr("127.0.0.1"); /* Check for server on loopback */ addr.sin_port=htons(29008); if(connect(cfd, (struct sockaddr *)&addr, sizeof(addr))<0) { perror("connect error"); return -1; } if(write(cfd, &ch, 1)<0) perror("write"); if(read(cfd, &ch, 1)<0) perror("read"); printf("nReply from Server: %cnn",ch); close(cfd); return 0; }
The flow of the client program is similar to the server. The first difference is that it specifies an Internet address for the server (the loop-back localhost address) in sin_addr
.
Next, instead of listening, it calls the connect()
system call to connect the socket referred to by sockfd
to the address specified by addr
. The returned descriptor will be used to communicate to the specified address.
Then, in the program, we have used write()
and read()
to send a character to the server, and receive a character, and then closed the descriptor.
Running the programs
Compile the programs as follows:
cc server.c -o server cc client.c -o client
Then, run them:
./server & ./client
Run each programs in a different terminal for a better view. See Figure 1 for the server’s output, and Figure 2 for the client’s.
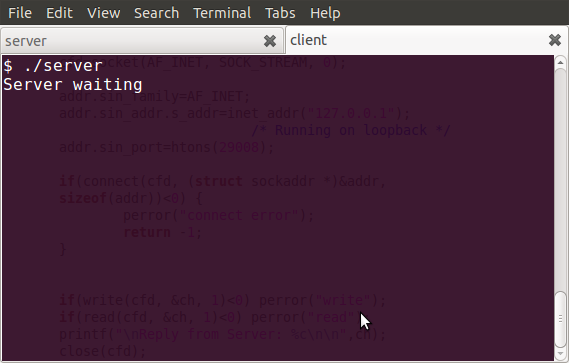
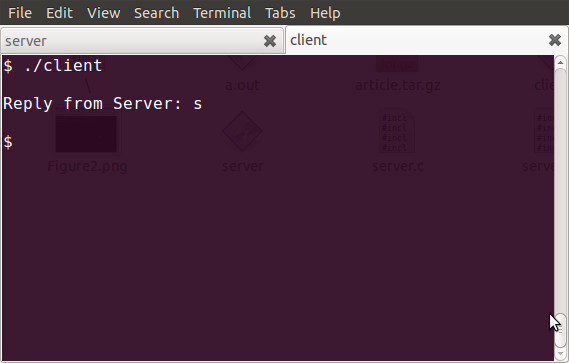
A good beginning, right? In the next article we’ll rewrite both these programs for IPv6, and move further to UDP. And yes, FOSS rocks!
[…] 2Written by Pankaj Tanwar on September 1, 2011 in Coding, Developers · 1 CommentEarlier, we created a simple server and client program using the socket API. This time, we’ll first start with a program, and then explain what’s going on. So […]
incase somebody has compiling issues or error like compilation termination use the code yum install glibc-devel.x86_64
yum install glibc-devel.x86_64
When I run this, the server never puts anything on the screen. The client works though… You have a typo: printf(“nReply from Server: %cnn”,ch);
It’s missing the backslash characters before the n’s.
I figured out that the reason the server never reported Waiting… was due to the missing /n on that line. Apparently, it doesn’t output until there’s a line break…