The ability to build apps is an important skill for a software developer. Building applications that run across multiple platforms, such as both Android and iOS, is important today. This article explores two tools in Python that help to build cross-platform apps—BeeWare and Kivy.
Apps are everywhere. One of the major reasons for the popularity of devices such as smartphones is the availability of innumerable apps. The ability to build apps is an important skill that software developers aim to acquire. As there are many platforms these days such as Android, iOS, Raspberry Pi, etc, developing applications that run across all of these has many benefits like consistency, singular code, etc. Hence, building cross-platform applications is getting popular among developers.
There are many languages to select from when you are developing an app. For example, if you want to develop an Android app, you have the following options:
- Java
- Kotlin
- Python
- C / C++ / C#
Apart from these, you can use Web technologies such as HTML, CSS and JavaScript as well to build mobile apps. The languages listed above cover only one platform — Android. Likewise, there are language options for each of the platforms such as Raspberry Pi and iOS.
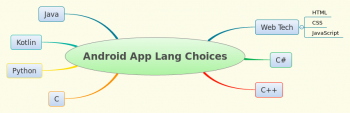
Selecting a particular language for development depends on various factors such as the nature of the application, the target user base and the expertise available. As per the IEEE Spectrum report, Python topped the language rankings list in 2019. Due to the availability of a large number of Python developers and the ease with which this language can be learned, it is a very good choice for app development with specialised frameworks and libraries.
This article focuses on building cross-platform apps. For this purpose, there are two important options in Python, as listed below:
- Kivy
- BeeWare
Both these frameworks have certain commonalities and differences as well. This article provides an introduction to their features and the steps involved in building your first app using these frameworks.
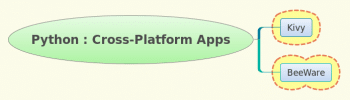
Kivy
Kivy is an open source Python library. It can be used for the rapid development of apps. It supports features such as multi-touch. The major features of Kivy are listed below:
- Cross-platform: Kivy supports the following platforms: Android, iOS, Raspberry Pi, Linux, OS X and Windows. The same code can be executed in all the supported platforms, which is a very useful feature for developers.
- Business-friendly: Kivy has no hidden costs. It is available with the MIT licence. Kivy is a stable framework and the documentation of the API is very good. Getting started with it is simple if you go through the official documentation.
- GPU accelerated: OpenGL ES2 is adapted to build the graphics engine.
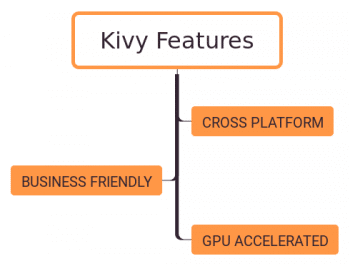
Kivy installation
Kivy can be installed on various platforms. Detailed installation instructions are available at https://kivy.org/#download. For example, if you are using conda, it can be done simply with the following command:
$ conda install kivy -c conda-forge
‘Hello World’ app in Kivy
Building a ‘Hello World’ application involves the following steps.
- Step 1: Create a derived class for the app class.
- Step 2: Implement the build method.
- Step 3: Create an instance of this class.
- Step 4: Call the run() method.
Sample code (from the official documentation) involving the above steps is shown below:
import kivy kivy.require(‘1.0.6’) # Use you kivy version here. from kivy.app import App from kivy.uix.label import Label class MyApp(App): def build(self): return Label(text=’Hello world’) if __name__ == ‘__main__’: MyApp().run()
The output for this Hello World app is shown in Figure 4.
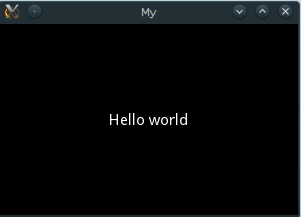
You can add more controls with the following code:
from kivy.app import App from kivy.uix.gridlayout import GridLayout from kivy.uix.label import Label from kivy.uix.textinput import TextInput class LoginScreen(GridLayout): def __init__(self, **kwargs): super(LoginScreen, self).__init__(**kwargs) self.cols = 2 self.add_widget(Label(text=’User Name’)) self.username = TextInput(multiline=False) self.add_widget(self.username) self.add_widget(Label(text=’password’)) self.password = TextInput(password=True, multiline=False) self.add_widget(self.password) class MyApp(App): def build(self): return LoginScreen() if __name__ == ‘__main__’: MyApp().run()
In this code, we have created a grid layout with two columns. Then, text input and label widgets are added for building a login screen.
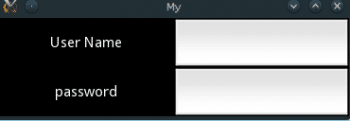
There is a gallery of applications available, which have been built with Kivy, at https://kivy.org/doc/stable/examples/gallery.html.
BeeWare
BeeWare works on the theme ‘Write once. Deploy everywhere’. BeeWare enables building apps in Python that can be released on the different platforms/environments listed below:
- Android
- iOS
- Linux
- Windows
- MacOS
- tvOS
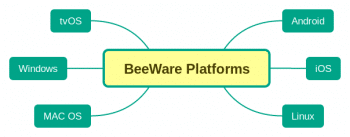
The primary objective of BeeWare is to enable the development of native user interfaces that are rich in nature, and are easier to develop and deploy across various types of devices. The goal of the BeeWare project is the development of tools to enable the following:
- Tools to package a Python project in such a manner that it can be executed on various types of devices.
- Providing libraries to access the native capabilities and widgets of these devices.
- Providing tools that facilitate the development, debugging and analysis of these projects.
The primary advantage of using BeeWare is that it uses the native widgets and native functionality in contrast to the theme based approach.
BeeWare is a collection of tools and libraries that work together to build cross-platform native GUI apps in Python. It includes the following:
- Toga: A widget toolkit that is supported across various platforms.
- Briefcase: A tool that enables the packaging of Python projects.
- Rubicon ObjC: A library for working with Objective C libraries from Python code. It is useful in iOS and MacOS.
- Rubicon Java: A library for working with Java from Python code.
These tools may be used individually or as a suite.
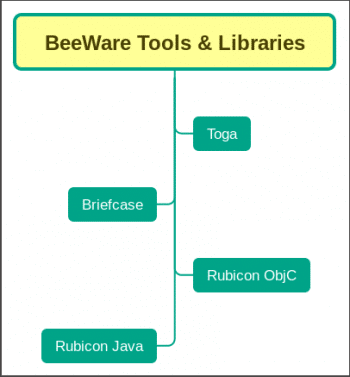
The first step is to install Briefcase, which can be done with the following command:
$ python -m pip install briefcase
After the successful installation of Briefcase, the first app can be created with the following command:
$ briefcase new
Briefcase will prompt you to enter the following details:
- Formal name: The default value can be accepted (Hello World)
- App name: Default value can be accepted (helloworld)
- Bundle
- Project name
- Description
- Author
- Author’s email
- URL: The landing page of your application
- Licence
- GUI framework: Here, the default option is Toga, which is BeeWare’s GUI toolkit, as explained earlier.
Based on the supplied values, the skeleton of the project is created, which will look similar to the one shown in Figure 8.
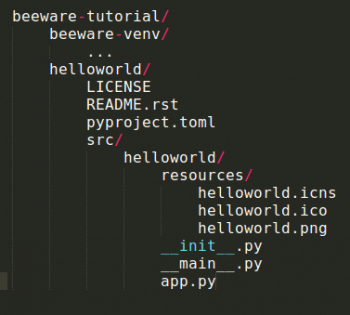
You can run this app in the developer mode with the following commands:
$ cd helloworld (beeware-venv) $ briefcase dev [hello-world] Installing dependencies... ... [helloworld] Starting in dev mode...
You will see the output shown in Figure 9.
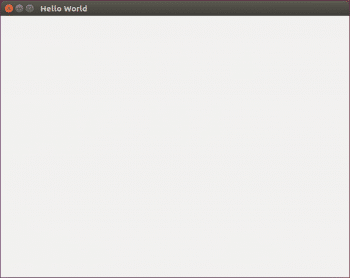
Tutorials for customising and deploying apps are available in the official documentation at https://docs.beeware.org/en/latest/index.html.
Kivy vs BeeWare
So far in this article, readers have been introduced to two Python based tools to build cross-platform apps — Kivy and BeeWare. Now let’s compare them.
- The most important difference between Kivy and BeeWare is the manner in which the widgets/controls are rendered. Kivy provides its own style in rendering widgets and hence they don’t look native. However, across the platforms, the applications look consistent. On the other hand, BeeWare uses the native user interface and hence the widgets/controls look native on the respective platforms in which they are running.
- BeeWare provides specialised tools such as Toga and Briefcase, which perform a specific task for which they are developed in an efficient manner.
- Kivy has been available since 2011, while BeeWare is relatively more recent. The latter is still evolving and has great potential in becoming the tool to build native apps in Python.
Both Kivy and BeeWare enable cross-platform app development in Python. Based on the nature of the application, either one of these tools can be selected. If you are a Python developer, give both Kivy and BeeWare a try to get the feel of cross-platform app development. In the coming years, we can expect these tools to evolve further and make Python based cross-platform development simpler and effective.