If there is anything that has caught the attention of the tech world in the last few weeks, it is ChatGPT. The media is projecting it as the next big thing after Google Search Engine, and experts are competing with each other in predicting its impact on society. This article tries to peek into ChatGPT from the perspective of a software developer.
ChatGPT is a chatbot backed by artificial intelligence. Built by San Francisco-headquartered OpenAI, it was announced to the world in the month of November 2022.
In the words of ChatGPT, it is an AI assistant trained to help answer questions and provide information on a wide range of topics, including general knowledge, history, science, mathematics, language, and many others. Sounds familiar? Are search engines already not doing that job? There is a difference!
According to OpenAI, ChatGPT has been trained to interact in a conversational way. The dialogue format makes it possible for ChatGPT to answer follow-up questions, admit its mistakes, challenge incorrect premises, and reject inappropriate requests.
Using ChatGPT
Well, let’s experience it! The first thing is to log in to ChatGPT. Visit the URL https://chat.openai.com/auth/login on any modern browser to open the login page. It appears as in Figure 1.
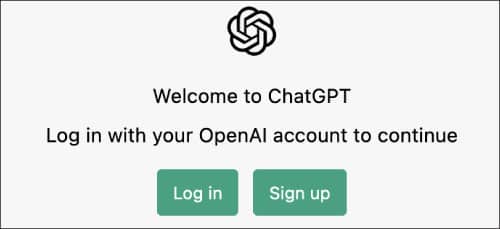
Since we haven’t signed up yet, click on the ‘Sign up’ button. It opens the dialog, offering multiple ways to sign up.
In my case, I chose to continue with Google. After entering the Google credentials, it prompted for a name and phone number to initiate the verification process. Upon entering the received OTP, the ChatGPT opened up at the URL https://chat.openai.com/chat, as in Figure 2.
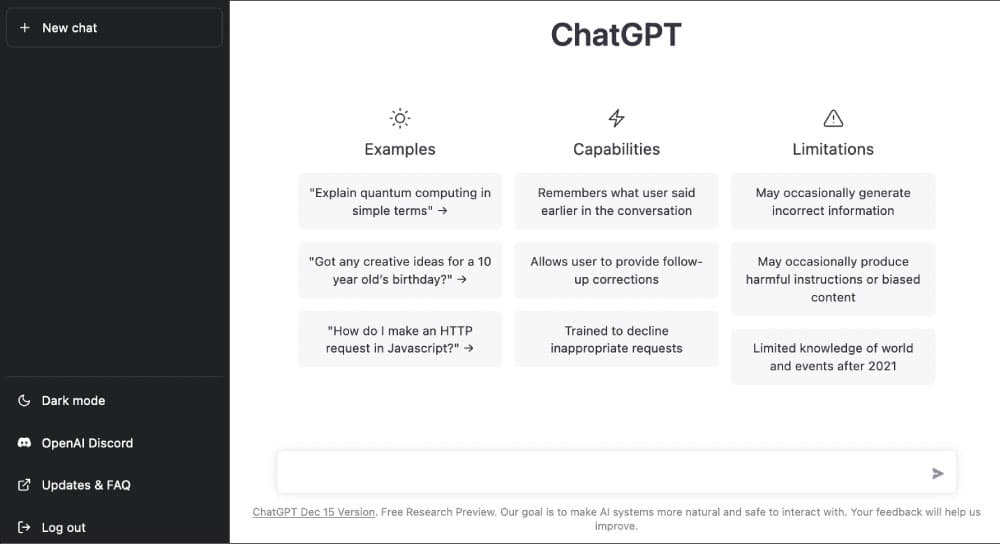
The ChatGPT clearly states that the current version is just a free research preview. Its goal is to get external feedback in order to improve its systems and make them safer. It also cautions that the system may occasionally generate incorrect or misleading information and produce offensive or biased content, despite the safeguards that are in place.
Since ChatGPT conversations may be reviewed by AI trainers to improve the systems, we must not share any sensitive information in our conversations.
Let’s play with ChatGPT
Programming is one of the areas in which ChatGPT proves to be a useful assistant. For instance, type the following question on the prompt and look at the response:
java command to compile a file
Apart from such simple questions, we can even ask ChatGPT to write code for us. How about getting some help in writing some Python code? I started a new conversation with the following prompt:
sort numbers using bubble sort in python
The assistant generated the following code:
def bubble_sort(numbers): n = len(numbers) for i in range(n): for j in range(0, n - i - 1): if numbers[j] > numbers[j + 1]: numbers[j], numbers[j + 1] = numbers[j + 1], numbers[j] # test the function numbers = [3, 4, 1, 2, 5] bubble_sort(numbers) print(numbers) # [1, 2, 3, 4, 5]
You can verify the code just by reviewing it in case you are a Python developer. Or copy the code into a source file, and try running it in a Python environment. It works!
But it doesn’t just end there. We know that there is more than one way to write the code for bubble sort. ChatGPT agrees. I asked her to try again.
This time it generated the following code:
def bubble_sort(numbers): # Set the flag to True to start the sorting flag = True # Keep looping until the flag is False while flag: # Set the flag to False flag = False # Loop through the numbers for i in range(len(numbers) - 1): # Check if the current number is greater than the next number if numbers[i] > numbers[i + 1]: # If it is, swap the numbers numbers[i], numbers[i + 1] = numbers[i + 1], numbers[i] # Set the flag to True to indicate that a swap has occurred flag = True # Return the sorted list return numbers sorted_numbers = bubble_sort([3, 5, 2, 1, 4]) print(sorted_numbers) # Output: [1, 2, 3, 4, 5]
You can keep asking it to try any number of times. In my case, the following was suggested in the third iteration:
def bubble_sort(numbers): # We set swapped to True so the loop looks runs at least once swapped = True while swapped: swapped = False for i in range(len(numbers) - 1): if numbers[i] > numbers[i + 1]: # Swap the elements numbers[i], numbers[i + 1] = numbers[i + 1], numbers[i] # Set the flag to True so we’ll loop again swapped = True # Verify it works numbers = [3, 7, 4, 9, 1, 6] bubble_sort(numbers) print(numbers) # Should print [1, 3, 4, 6, 7, 9]
Tired of different versions of the code for the same problem? How about asking the assistant to optimize the code instead? I just typed the following on the prompt:
optimize
The following is the result.
There are several ways you can optimize the bubble sort algorithm to make it run faster. Here are a few ideas: Use a flag to keep track of whether any swaps were made on the last iteration of the loop. If no swaps were made, it means the list is already sorted and we can exit the loop early. Only iterate up to the last element that has already been sorted. On each pass, the largest element will “bubble” up to the end of the list, so there’s no need to compare it to any other elements on the next pass. Use a different sorting algorithm altogether, such as quicksort or merge sort, which have better average-case time complexity than bubble sort. I hope these suggestions are helpful! Let me know if you have any questions.
I am sure you noticed that the assistant is remembering the whole conversation and answering the questions contextually.
Development with ChatGPT
The above conversation only illustrates how the assistant can help us in writing code for smaller problems. However, developing software is not just about writing code. Let’s act as if we do not know much about developing Spring Boot applications and see how much the assistant helps us.
I started by asking the following question:
difference between log4j and slf4j
It gave a whole lot of information. Try this yourself and find out how useful it is.
Then, I asked the following question:
spring program with slf4j
It generated the following code:
import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class MySpringApp { private static final Logger logger = LoggerFactory.getLogger(MySpringApp.class); public static void main(String[] args) { SpringApplication.run(MySpringApp.class, args); logger.info(“Spring application started!”); } }
The assistant does not wash its hands off with just code. It gives a lot of notes as well. For instance, the following is the note generated along with the above code.
In this example, we use the LoggerFactory class from slf4j to create a logger for the MySpringApp class. We can then use the logger object to output log statements at different levels (e.g., info, debug, error). To use slf4j in a Spring application, you will need to include the slf4j-api library as a dependency in your project. You will also need to include a concrete implementation of slf4j, such as log4j, logback, or java.util.logging. For example, to use log4j as the underlying logging implementation, you will need to include the log4j library as a dependency in your project. You can then configure log4j to output log statements to the desired destination (e.g., console, file).
Since it is advising some dependencies, I acted as if I do not know how it can be done. So, I asked this follow-up question:
pom file for spring with slf4j
Here is the resulting suggestion:
<project> <!-- Other project configuration --> <dependencies> <!-- Other dependencies --> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-api</artifactId> <version>1.7.30</version> </dependency> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-log4j12</artifactId> <version>1.7.30</version> </dependency> </dependencies> </project>
Again, the notes suggested a log4j dependency. I asked the assistant to add that too, and it generated the following refined configuration.
<project> <!-- Other project configuration --> <dependencies> <!-- Other dependencies --> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-api</artifactId> <version>1.7.30</version> </dependency> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-log4j12</artifactId> <version>1.7.30</version> </dependency> <dependency> <groupId>log4j</groupId> <artifactId>log4j</artifactId> <version>1.2.17</version> </dependency> </dependencies> </project> You will also need to include a log4j.properties file in your project to configure log4j to output log statements to the desired destination (e.g., console, file).
The story doesn’t end here. I asked the assistant to help me with the log4j.properties file:
log4j.properties for spring with slf4j
It obliged.
# Root logger log4j.rootLogger=INFO, stdout, file # Console appender log4j.appender.stdout=org.apache.log4j.ConsoleAppender log4j.appender.stdout.layout=org.apache.log4j.PatternLayout log4j.appender.stdout.layout.ConversionPattern=%d{yyyy-MM-dd HH:mm:ss} %-5p %c{1}:%L - %m%n # File appender log4j.appender.file=org.apache.log4j.FileAppender log4j.appender.file.File=app.log log4j.appender.file.layout=org.apache.log4j.PatternLayout log4j.appender.file.layout.ConversionPattern=%d{yyyy-MM-dd HH:mm:ss} %-5p %c{1}:%L - %m%n
I was thrilled. Not that we cannot get this help from the web using a search engine. But the conversational experience with ChatGPT was just awesome.
Let us try ChatGPT for one last time by taking help in developing and deploying REST API. I started a new conversation with a simple question:
design rest api to add a customer
It responded with the following explanation:
To design a REST API to add a customer, you will need to define the endpoints and the request/response payloads for the API. Here is an example of how you could design the API: Endpoint: POST /customers Request payload: { “name”: “John Smith”, “email”: “john@example.com”, “phone”: “555-555-5555” }
Quite useful! We normally use Swagger for documenting the REST API. There is a lot of boilerplate in it. ChatGPT is perfect in such cases. I asked the following in the same conversation:
swagger doc for adding a new customer
Here is Swagger documentation for the POST /customers endpoint that I described above:
/customers: post: summary: Add a new customer requestBody: content: application/json: schema: type: object required: - name - email - address properties: name: type: string email: type: string format: email address: type: string responses: 201: description: The customer was successfully created content: application/json: schema: type: object properties: id: type: integer format: int64 name: type: string email: type: string format: email address: type: string 400: description: The request body is invalid 409: description: A customer with the same email already exists
What else could I ask for? The final version of the documentation may not look this simple. Yet, it is a good starting point.
I was curious to see if ChatGPT could give me code for publishing the above REST API using Spring Boot. It did! The question was this:
spring controller for the above endpoint
And the response was:
@RestController @RequestMapping(“/customers”) public class CustomerController { @PostMapping public ResponseEntity<Customer> createCustomer(@Valid @RequestBody Customer customer) { // save the customer and return it Customer savedCustomer = customerRepository.save(customer); return ResponseEntity.status(HttpStatus.CREATED).body(savedCustomer); } }
This is imperative Spring. Nowadays, we prefer the reactive paradigm. I asked the same thing with this question:
i want it with spring reactive
ChatGPT gave the starter code.
@RestController @RequestMapping(“/customers”) public class CustomerController { @PostMapping public Mono<ResponseEntity<Customer>> createCustomer(@Valid @RequestBody Mono<Customer> customer) { // save the customer and return it return customer.flatMap(customer -> customerRepository.save(customer)) .map(savedCustomer -> ResponseEntity.status(HttpStatus.CREATED).body(savedCustomer)); } }
No modern development is completed without creating a Docker image. I took help from ChatGPT to generate a Dockerfile for the above code with this question:
dockerfile for the above application
And I got the following response, which is sufficient to start with:
FROM openjdk:11-jdk-slim ARG JAR_FILE=target/*.jar COPY ${JAR_FILE} app.jar EXPOSE 8080 ENTRYPOINT [“java”, “-jar”, “app.jar”]
Instead of asking for specific help, I just tried the following to spice up the conversation:
what is the next step?
It surprised me with this answer:
Once you have written the code for your application and created a Dockerfile, the next step is to build a Docker image and run a Docker container. To build the Docker image, open a terminal and navigate to the directory containing the Dockerfile. Then, run the following command: docker build -t my-app . This will build a Docker image with the name “my-app” using the instructions in the Dockerfile. To run a Docker container, use the following command: docker run -p 8080:8080 my-app This will start a Docker container from the “my-app” image, and map the container’s 8080 port to the host’s 8080 port. Is there anything else I can help with?
See! ChatGPT maintains the conversational context. It answers in the given context, not just dry static results. And that is amazing. What else can I say? I said:
thanks
And the assistant was good enough to acknowledge.
You’re welcome! I’m glad I could help. If you have any other questions, feel free to ask. I’ll do my best to assist you.
ChatGPT: Boon or Bane?
I remember people arguing about the good and bad of the computerisation of banks in the 90s and autonomous vehicles in the 2020s. Every disruptive technology raises this question. It’s the same with ChatGPT as well.
The precise question that is relevant for the software development community is this: Will ChatGPT replace developers?
At this point, the answer is clearly ‘no’. Technology always destroys some jobs and creates new ones. But replacing a developer takes a lot of effort. Software development is not just coding. Certainly, ChatGPT can help in writing code just like IDE helps in code completion, compilation, debugging, etc. But, like IDE, it cannot replace human involvement in end-to-end development. We, as developers, used text editors to write the code in the 80s, and used IDEs in the 90s. We have copied code from Google search results and used solutions from StackOverflow. All of this was done to improve productivity. And yet, none of them were really able to replace the developer. The same is going to happen with ChatGPT as well. It’s a disruptive productive tool for programmers. But, it neither makes you a programmer nor takes away your programming job. At least for now!