Here’s an overview of the Processing programming language, and a tutorial to demystify the process of building a simple animation with it.
In the digital era, animation can do more than just entertain children. It’s an effective tool for visual communication. Of course, it offers a whole new medium for expression and creativity, but at a more practical level, the movement in an animation attracts more attention than static images.
Programming can be fun and a very creative activity. Playing with graphics and sound is a great way to start programming. Let’s answer three simple questions to get started: What is Processing, who should use it, and why should they do so?
The Processing programming language
Processing is an open source graphical library and integrated development environment built for the creation of digital arts and multimedia, with the purpose of teaching non-programmers the fundamentals of computer programming in a visual context.
It is based on Java and all the code gets pre-processed and converted directly into Java code when you hit the Run button. Java’s PApplet class is the base class for all Processing sketches.
Processing is easy to learn, without having to type a lot of code. It is so simple that you can read through the code and get a rough idea of the program flow.
Who can use Processing?
Processing is designed not only for coders who want to create interactive visual programs but can also be used by designers and artists with minimal, if any, programming experience. If that sounds interesting to you, then welcome to Processing!
The features of Processing are:
- Free to download and is open source
- Interactive programs with 2D, 3D, PDF, or SVG
- Easy to learn and understand
- Available for GNU/Linux, Mac OS X, Windows, Android and ARM
- Over 100 libraries to extend the core software
Processing consists of:
- Processing Development Environment (PDE): The PDE is an Integrated Development Environment (IDE) with a minimalist set of features.
- A collection of functions (also referred to as commands or methods) that make up the ‘core’ programming interface.
- A language syntax, identical to Java but with a few modifications.
- An active online community.
Ready to code? Let’s get our hands dirty!
Download Processing from the link. On Windows, when you click on the link, a .zip file will be downloaded. Double-click it to extract the files and click Processing.exe to start (https://download.processing.org/processing-3.5.4-windows64.zip).
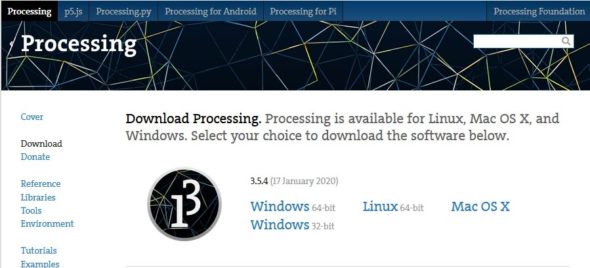
The Processing equivalent of a ‘Hello World’ program is simply to draw a line:
line (1,2,5,2)
This code calls Processing’s line function, which tells the computer to draw a line.
This line of code means, ‘Draw a line, from coordinate (1,2) to (5,2)’. Line of code represents a line (x1, y1, x2, y2) where (x1, y1) are coordinates of the first point, and (x2, y2) are the coordinates of the second point. Click Run (the triangle button in the toolbar), and your code gets compiled and executed. By nature, Processing programs never get terminated; they run forever and ever until they get disturbed. You can terminate them programmatically; however, if you don’t, you can use the Stop button. You’ve used the Processing editor to write your first line of code. Congratulations!
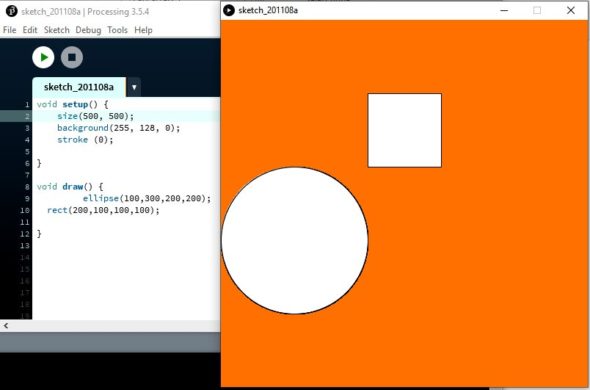
Now, let’s understand the structure of the program in Processing. Processing code consists of two main parts, setup() and draw() blocks. The setup block runs once when the code gets executed, and the draw blocks run continuously.
void setup() { size(500, 500); background(255, 128, 0); stroke (0); } void draw() { ellipse(100,300,200,200); rect(200,100,100,100); }
There are dozens of different functions to display geometric shapes in Processing. I will now use two of them: rect() and ellipse(). This version sets the window size to 500×500 pixels, background to bright orange, and draws a rectangle and ellipse in white by setting the stroke colour to black.
The problem with objects drawn so far is that they are static. How do we make them move?
The answer is simple! It’s by using variables to increment/decrement coordinates.
Variables are names that hold values. To create a variable, you give it a type, a name, and a value.
a) The type tells Processing about the kind of value the variable will hold.
b) The name is how you’ll use the variable later in the code.
c) The value is what the variable points to.
For example, int a =15. This line of code creates an integer variable a that points to value 15.
Let’s try to create a simple moving line animation with the help of variables.
int a = 0; void setup() { size(640, 360); stroke(255); background(0); } void draw() { background(0); a = a + 2; line(a, 0, a, height/2); if(a > width) { a = 0; } }
Let’s try to decrypt the code:
1. Int a=0 denotes an integer variable with the name ‘a’ and value 0.
2. Size (640, 360) defines the window’s size (width, height).
3. Stroke(255) sets the stroke colour to objects. Stroke property is applicable for lines and borders around objects.
4. I have mentioned the background(0) in the draw block; otherwise, each time the draw block iterates, ‘a’ increases by two, and therefore the line gets redrawn to two pixels. However, the lines drawn from the previous iterations remain in the view.
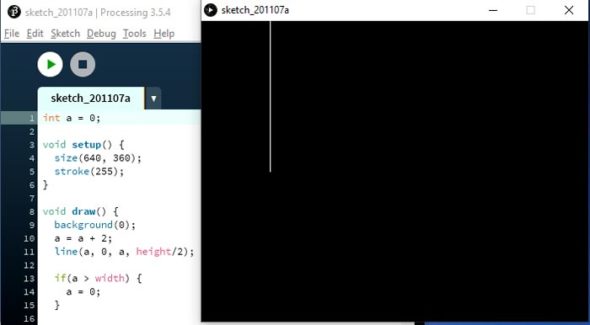
To get rid of the marks the line leaves behind, we simply remove the background(0) from the setup block and paste it to be the very first line of the draw block. When background code is in the setup block, it runs once at the beginning, making the background black. But this isn’t enough; we need the background to be set to black in each loop to cover the lines drawn from the previous loops. The background, being in the first line, runs first and becomes the base layer. On each loop, the canvas is painted black and new elements get drawn on top of the black background. So, we have no marks.
Now, let’s move on to the most important part of the code:
a = a + 2; line(a, 0, a, height/2); if(a > width) { a = 0; }
i. The first line of code is an increment operator, where the value of ‘a’ increases with every iteration.
ii. The second line states that with every increment in the value of a, draw a line from the starting point (0, 0). Its height is half the length of the canvas.
The increment happens in the x coordinate like 0, 2, 4, ……., while the y coordinate remains the same.
iii. In the last part of the code, for the if block, we have defined a condition that if the length of the line is equal to the width of the canvas, then rather than disappearing, the line will move again from its starting point because we have changed the counter value of a to 0.
The idea behind animating things in Processing is manipulating the object’s coordinates programmatically to change their location.